- A Map is an object that maps keys to values.
- A map cannot contain duplicate keys.
- Each key can map to at most one value.
- It models the mathematical function abstraction.
A map contains values on the basis of key, i.e. key and value pair. Each key and value pair is known as an entry. A Map contains unique keys.
A Map is useful if you have to search, update or delete elements on the basis of a key.
Note:
The Map
interface maintains 3 different sets:
- the set of keys
- the set of values
- the set of key/value associations (mapping).
Interface Map<K,V>
- Type Parameters:
K
– the type of keys maintained by this mapV
– the type of mapped values
public interface Map<K,V>
- An object that maps keys to values. A map cannot contain duplicate keys; each key can map to at most one value.
- This interface takes the place of the Dictionary class, which was a totally abstract class rather than an interface.
- The Map interface provides three collection views, which allow a map’s contents to be viewed as a set of keys, collection of values, or set of key-value mappings.
public static interface Map.Entry<K,V>
- A map entry (key-value pair).
- The Map.entrySet method returns a collection-view of the map, whose elements are of this class.
ARRAYS LIST:
- Arraylist class implements List interface and it is based on an Array data structure.
- It is widely used because of the functionality and flexibility it offers.
- Most of the developers choose Arraylist over Array as it’s a very good alternative of traditional java arrays.
- ArrayList is a resizable-array implementation of the
List
interface. - It implements all optional list operations, and permits all elements, including
null
.
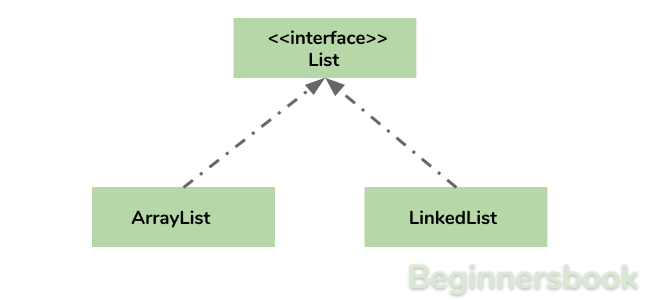
Why ArrayList is better than Array?
The limitation with array is that it has a fixed length so if it is full you cannot add any more elements to it, likewise if there are number of elements gets removed from it the memory consumption would be the same as it doesn’t shrink.
On the other ArrayList can dynamically grow and shrink after addition and removal of elements (See the images below). Apart from these benefits ArrayList class enables us to use predefined methods of it which makes our task easy. Let’s see the diagrams to understand the addition and removal of elements from ArrayList and then we will see the programs.
Adding Element in ArrayList at specified position:
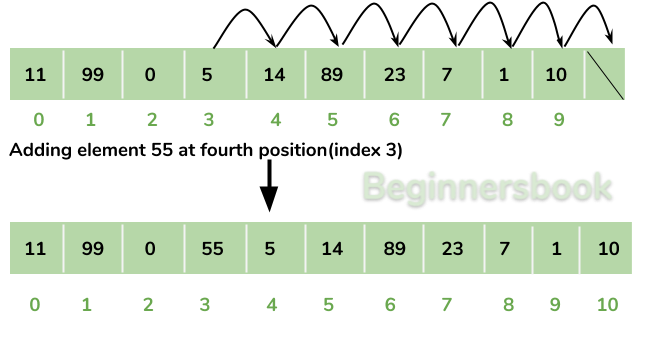
Removing Element from ArrayList:
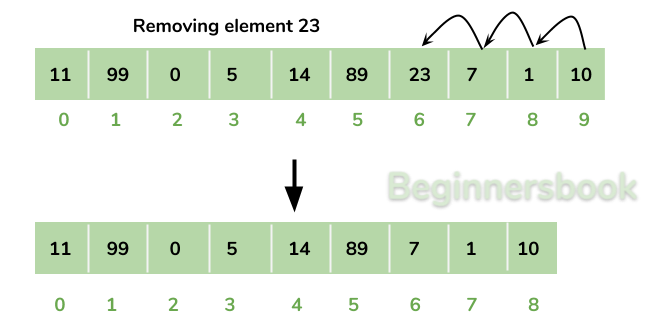
Methods of ArrayList class:
There are number of methods available which can be used directly using object of ArrayList class. Let’s discuss few important methods of ArrayList class.
1) add( Object o):
This method adds an object o to the arraylist.
obj.add("hello");
This statement would add a string hello in the arraylist at last position.
2) add(int index, Object o):
It adds the object o to the array list at the given index.
obj.add(2, "bye");
It will add the string bye to the 2nd index (3rd position as the array list starts with index 0) of array list.
3) remove(Object o):
Removes the object o from the ArrayList.
obj.remove("Chaitanya");
This statement will remove the string “Chaitanya” from the ArrayList.
4) remove(int index):
Removes element from a given index.
obj.remove(3);
It would remove the element of index 3 (4th element of the list – List starts with o).
5) int size():
It gives the size of the ArrayList – Number of elements of the list.
6) clear():
It is used for removing all the elements of the array list in one go. The below code will remove all the elements of ArrayList whose object is obj.
obj.clear();
REFERENCE LINK:https://beginnersbook.com/2013/12/java-arraylist/
SAMPLE PROGRAM:
import java.util.ArrayList;
import java.util.List;
public class Avoid
{
public static void main(String[] args)
{
ArrayList al = new ArrayList();
al.add(5);
al.add(“Raja”);
al.add(5.4f);
al.add(true);
//System.out.println(al);
//System.out.println(al.contains(5.4f));
//System.out.println(al.contains(5.3f));
//al.clear();
//System.out.println(al);
//System.out.println(al.indexOf(true));
//System.out.println(al.get(1));
//System.out.println(al.getClass());
//System.out.println(al.isEmpty());
//al.clear();
//System.out.println(al.isEmpty());
ArrayList al2 = new ArrayList();
al2.addAll(al);
System.out.println(al2);
ArrayList al3 = new ArrayList();
al3.add(100);
al3.add(200);
al3.add(300);
al2.addAll(al3);
System.out.println(al2);
//al2.addAll(2, al3);//method overloading
//System.out.println(al2);
al2.add(3,457);
System.out.println(al2);
//al2.remove(0);
//al2.remove(true);
//al2.removeAll(al);
al2.retainAll(al);
List al4 = al2.subList(1,2);
System.out.println(al4);
System.out.println(al);
//al.remove(0);
//System.out.println(al);
//al.remove(“Raja”);
//System.out.println(al);
}
}
/*OUTPUT:
[5, Raja, 5.4, true]
[5, Raja, 5.4, true, 100, 200, 300]
[5, Raja, 5.4, 457, true, 100, 200, 300]
[Raja]
[5, Raja, 5.4, true]
*/
LinkedList:
Similar to arrays in Java, LinkedList is a linear data structure. However LinkedList elements are not stored in contiguous locations like arrays, they are linked with each other using pointers. Each element of the LinkedList has the reference(address/pointer) to the next element of the LinkedList.
LinkedList representation:
Each element in the LinkedList is called the Node. Each Node of the LinkedList contains two items: 1) Content of the element 2) Pointer/Address/Reference to the Next Node in the LinkedList.
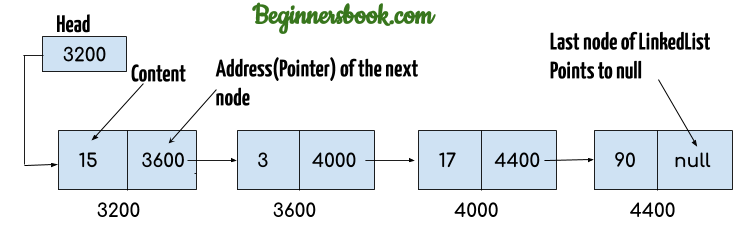
Note:
1. Head of the LinkedList only contains the Address of the First element of the List.
2. The Last element of the LinkedList contains null in the pointer part of the node because it is the end of the List so it doesn’t point to anything as shown in the above diagram.
3. The diagram which is shown above represents a singly linked list. There is another complex type variation of LinkedList which is called doubly linked list, node of a doubly linked list contains three parts: 1) Pointer to the previous node of the linked list 2) content of the element 3) pointer to the next node of the linked list.
Why do we need a Linked List?
1) Size of the array is fixed.
2) Array elements need contiguous memory locations to store their values.
3) Inserting an element in an array is performance wise expensive as we have to shift several elements to make a space for the new element.
Similarly deleting an element from the array is also a performance wise expensive operation because all the elements after the deleted element have to be shifted left.
REFERENCE LINK:https://beginnersbook.com/2013/12/linkedlist-in-java-with-example/
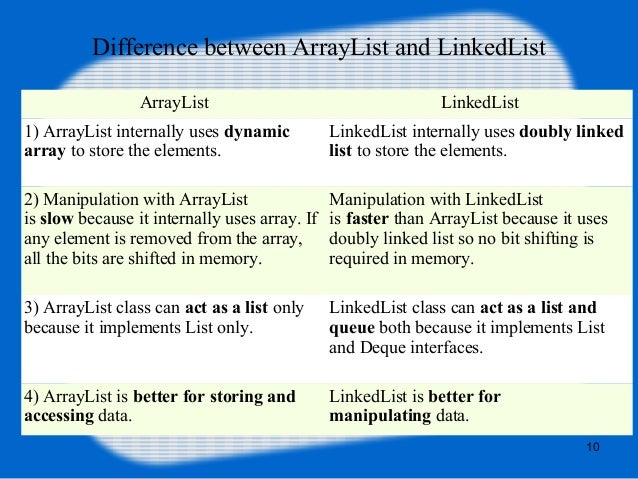

SAMPLE PROGRAM:
import java.util.ArrayList;
import java.util.List;
public class Avoid
{
public static void main(String[] args)
{
ArrayList al = new ArrayList();
al.add(5);
al.add(“Raja”);
al.add(5.4f);
al.add(true);
//System.out.println(al);
//System.out.println(al.contains(5.4f));
//System.out.println(al.contains(5.3f));
//al.clear();
//System.out.println(al);
//System.out.println(al.indexOf(true));
//System.out.println(al.get(1));
//System.out.println(al.getClass());
//System.out.println(al.isEmpty());
//al.clear();
//System.out.println(al.isEmpty());
ArrayList al2 = new ArrayList();
al2.addAll(al);
System.out.println(al2);
ArrayList al3 = new ArrayList();
al3.add(100);
al3.add(200);
al3.add(300);
al2.addAll(al3);
System.out.println(al2);
//al2.addAll(2, al3);//method overloading
//System.out.println(al2);
al2.add(3,457);
System.out.println(al2);
//al2.remove(0);
//al2.remove(true);
//al2.removeAll(al);
al2.retainAll(al);
List al4 = al2.subList(1,2);
System.out.println(al4);
System.out.println(al);
//al.remove(0);
//System.out.println(al);
//al.remove(“Raja”);
//System.out.println(al);
}
}
/*OUTPUT:
[5, Raja, 5.4, true]
[5, Raja, 5.4, true, 100, 200, 300]
[5, Raja, 5.4, 457, true, 100, 200, 300]
[Raja]
[5, Raja, 5.4, true]
*/